Get solid hands-on experience with Kubernetes and be able to deploy any application into production using Kubernetes Part 2
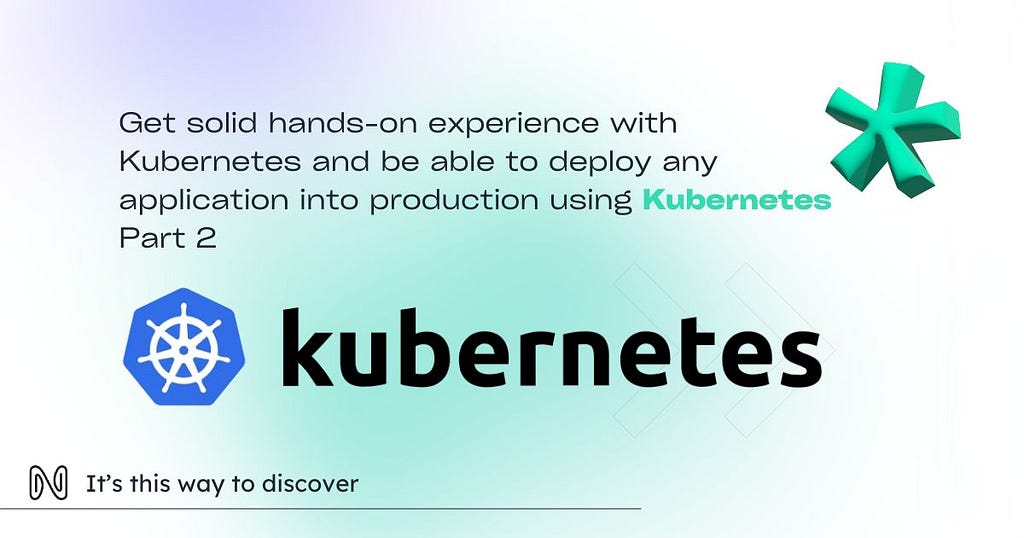
About This Article
- Create express app and deploy it using declarative approach.
- Learn how to communicate two pods in the same node.
Before starting this article be sure that you have read the first article it allow you to deploy simple FastAPI web app using declarative approach:
Get solid hands-on experience with Kubernetes and be able to deploy any application into production…
1 — Let’s move on building the express app :
A — Create directory k8s-web-hello
B —cd k8s-web-hello & npm init
D — npm install express
E — Open index.js and edit it with the code below and please change the name of the file to index.mjs which implement two api’s :
import express from 'express'
const app = express()
const PORT = 3000
app.get(
"/", (req, res) => {
const helloMessage = 'Message : Hello k8s trainer '
console.log(helloMessage)
res.send(helloMessage)
}
)
app.get(
"/fastapi", (req, res) => {
const helloMessage = 'Welcome to express from fastapi request'
console.log(helloMessage)
res.send(helloMessage)
}
)
app.listen(PORT ,() => {
console.log(`Web app is listening at the port ${PORT}`)
})
F — Finally edit package.json scripts part :
"scripts": {
"start": "node index.mjs"
},
Now let’s move on to dockerize the app and test it locally .
Create a Dockerfile or on terminal vim Dockerfile and paste the code below .
FROM node:alpine
WORKDIR /app
EXPOSE 3000
COPY package.json package-lock.json ./
RUN npm install
COPY . ./
CMD ["npm", "start"]
After adding everything be sure your repository have to be like that:
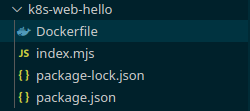
Let’s build the image :
docker build . -t khaledhadjali/k8s-web-hello
Note : change khaledhadjali to your docker hub id .
This is how to run the image locally :
docker run -p 3000:3000 -i -t khaledhadjali/k8s-web-hello:latest

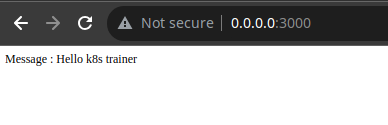
You can use the image from my docker hub directly .
After building and testing the image we move to push it to docker hub .
First be sure that u have logged in to docker hub using terminal via :
docker login
Then Push your image to your docker hub account:
docker push khaledhadjali/k8s-web-hello
Open your docker hub account and you have to find the image that you have pushed, otherwise you may use my public image in this link https://hub.docker.com/r/khaledhadjali/k8s-web-hello .
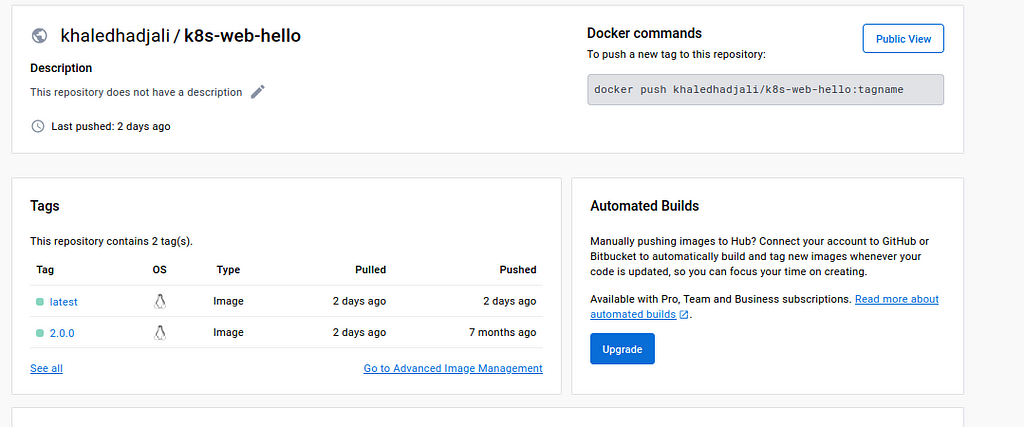
We have finished creating the express web application ,building docker image and pushing it to the docker hub .
We move to the part of deployment using k8s .
Deployment part :
Create a file and name it deployment and paste the code below which container the service and the deployment .
apiVersion: v1
kind: Service
metadata:
name: k8s-web-hello
spec:
type: LoadBalancer
selector:
app: k8s-web-hello
ports:
- port: 3000
targetPort: 3000
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: k8s-web-hello
spec:
replicas: 1
selector:
matchLabels:
app: k8s-web-hello
template:
metadata:
labels:
app: k8s-web-hello
spec:
containers:
- name: k8s-web-hello
image: khaledhadjali/k8s-web-hello
resources:
limits:
memory: "128Mi"
cpu: "500m"
ports:
- containerPort: 3000
Let’s apply our deployment :
kubectl apply -f deployment.yml
How to check that our pod is running :
kubectl get pods

On the Ready column it may be on 0/1 just wait a while and it will be 1/1 just it takes time to pull the image .
to see the result on your browser please write the command below :

And this is the result on the browser

Now we will modify the FastAPI app that we have built last time :
First we modify the requirements.txt by adding new package : requests .
fastapi
uvicorn
requests
Then we modify the app.py by implementing the express api that communicate with the api deployed in the other express pod :
from fastapi import FastAPI
import uvicorn
import requests
app = FastAPI()
@app.get("/")
def norma_root():
return { "Message": "Welcome to python" }
# this is the api that communicate with express api
@app.get("/express")
def express():
resp = requests.get('http://k8s-web-hello:3000/fastapi')
return {'res':resp.text}
if __name__ == '__main__':
uvicorn.run(app, host='0.0.0.0', port=8000)
In the second API express when we request the API we put the name of the service k8s-web-hello rather than localhost
Then build and push the image :
docker build . -t khaledhadjali/k8s-hello-fastapi
docker push khaledhadjali/k8s-hello-fastapi
Deploy the image using kubectl command:
kubectl delete -f k8s-web-fastapi.yml
kubectl apply -f k8s-web-fastapi.yml
To see the result on your browser please write the command below :


Let’s access our api that communicate with express :

And u may check the log of the pod like in the image below :
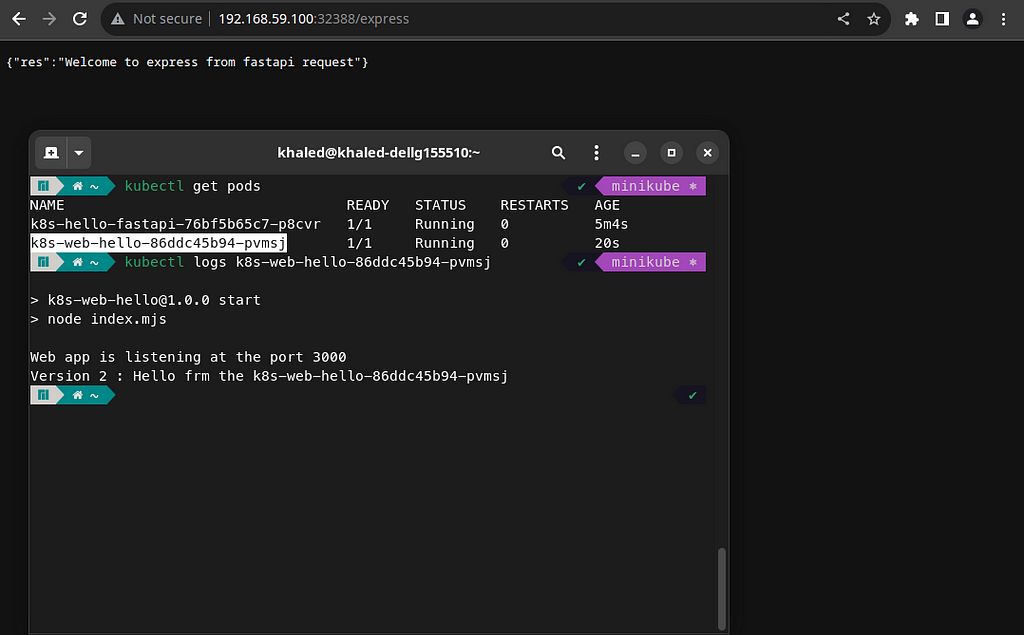
First you need to get all the pods and apply the command :
kubectl get pods
kubectl logs pod_name
TIPS:
The last part is how to enter container shell and use CURL command :
kubectl exec -it pod_name command_shell
kubectl exec -it k8s-hello-fastapi-76bf5b65c7-p8cvr sh

Install CURL command:
apk add curl
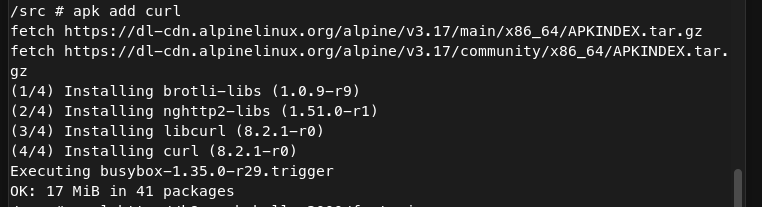
Execute curl command:
curl http://k8s-web-hello:3000/fastapi

Finally u may found all the codes in my GitHub repository :
https://github.com/khaled-ha/quick_intro_k8s
Conclusion
In this comprehensive guide, we’ve successfully navigated through building an Express application, dockerizing it, testing locally, and deploying it using Kubernetes. Moreover, we enhanced a FastAPI application to communicate with our Express service deployed in another pod.
This hands-on approach solidifies our understanding and capability of deploying applications into production using Kubernetes. By leveraging Docker and Kubernetes, we ensure scalable, reliable, and efficient application deployments. Remember, for a holistic understanding, ensure you’ve gone through Part 1 of this series. Stay updated and optimize your cloud-native journey with Kubernetes!